Components documentation
This page contains an overview + examples on all available components on the current solution. The code for the components are found in /Templates/Designs/Rapido/Components.
How to overwrite existing components
How to write your own components
The detailed Components documentation
The detailed Blocks documentation
Helper method: RenderButton
Settings
ConfirmText | System.String |
ConfirmTitle | System.String |
ButtonType |
|
Link | System.String |
Target |
|
IconPosition |
|
Id | System.String |
Title | System.String |
AltText | System.String |
OnClick | System.String |
CssClass | System.String |
Disabled | System.Boolean |
Icon |
Component {
Prefix
Name
Color
CssClass
LabelPosition
Label
HelperName
}
|
Name | System.String |
Href | System.String |
ButtonLayout |
|
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Button { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Button { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderCheckboxField
Settings
Checked | System.Boolean |
Type |
|
Name | System.String |
Id | System.String |
Label | System.String |
HelpText | System.String |
Link |
Component {
Target
Rel
Download
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Value | System.String |
Disabled | System.Boolean |
Required | System.Boolean |
ErrorMessage | System.String |
CssClass | System.String |
OnChange | System.String |
OnClick | System.String |
WrapperCssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new CheckboxField { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new CheckboxField { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderCheckboxListField
Settings
Options |
Component {
Capacity
Count
Item
}
|
Name | System.String |
Id | System.String |
Label | System.String |
HelpText | System.String |
Link |
Component {
Target
Rel
Download
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Value | System.String |
Disabled | System.Boolean |
Required | System.Boolean |
ErrorMessage | System.String |
CssClass | System.String |
OnChange | System.String |
OnClick | System.String |
WrapperCssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new CheckboxListField { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new CheckboxListField { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderContentModule
Add the module as a text string
Settings
Content | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ContentModule { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ContentModule { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderDashboard
Settings
WidgetsBaseBackgroundColor | System.String |
CssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Dashboard { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Dashboard { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderDashboard
Settings
WidgetsBaseBackgroundColor | System.String |
CssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Dashboard { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Dashboard { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderDashboardWidgetCounter
Settings
Count | System.Int32 |
Icon |
Component {
Prefix
Name
Color
CssClass
LabelPosition
Label
HelperName
}
|
Title | System.String |
BackgroundColor | System.String |
CssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new DashboardWidgetCounter { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new DashboardWidgetCounter { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderDashboardWidgetLink
Settings
Link | System.String |
Icon |
Component {
Prefix
Name
Color
CssClass
LabelPosition
Label
HelperName
}
|
Title | System.String |
BackgroundColor | System.String |
CssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new DashboardWidgetLink { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new DashboardWidgetLink { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderFileField
Settings
ChooseFileText | System.String |
NoFilesChosenText | System.String |
UploadButton |
Component {
ConfirmText
ConfirmTitle
ButtonType
Link
Target
IconPosition
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Multiple | System.Boolean |
Name | System.String |
Id | System.String |
Label | System.String |
HelpText | System.String |
Link |
Component {
Target
Rel
Download
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Value | System.String |
Disabled | System.Boolean |
Required | System.Boolean |
ErrorMessage | System.String |
CssClass | System.String |
OnChange | System.String |
OnClick | System.String |
WrapperCssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new FileField { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new FileField { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderForm
Settings
Name | System.String |
Method |
|
Action | System.String |
Enctype |
|
OnSubmit | System.String |
CssClass | System.String |
FormStartMarkup | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Form { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Form { OPTIONAL_settings })
Markup result
Helper method: RenderHeading
My new heading
Settings
Title | System.String |
Level | System.Int32 |
Color | System.String |
Link | System.String |
CssClass | System.String |
Icon |
Component {
Prefix
Name
Color
CssClass
LabelPosition
Label
HelperName
}
|
IconPosition |
|
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Heading { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Heading { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderHiddenField
Settings
Value | System.String |
Id | System.String |
Name | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
Label | System.String |
Disabled | System.Boolean |
Required | System.Boolean |
ErrorMessage | System.String |
CssClass | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new HiddenField { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new HiddenField { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderIcon
Icon label
Settings
Prefix | System.String |
Name | System.String |
Color | System.String |
CssClass | System.String |
LabelPosition |
|
Label | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Icon { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Icon { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderImage
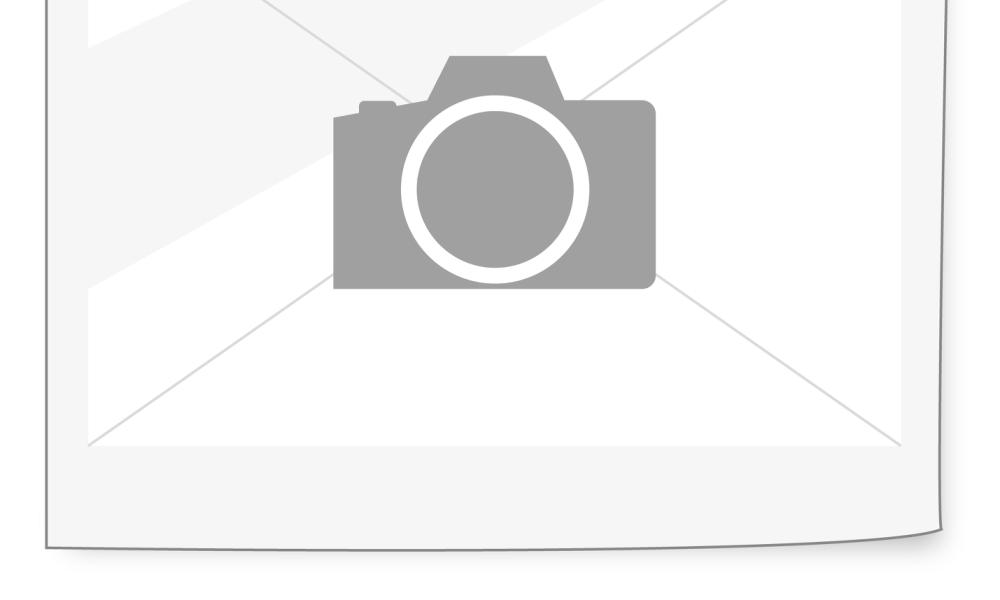
Settings
Path | |
Title | System.String |
Id | System.String |
Link | System.String |
OnClick | System.String |
Caption | System.String |
CssClass | System.String |
Style |
|
ImageDefault |
Component {
Width
Height
Crop
DoNotUpscale
FillCanvas
Compression
Quality
Format
BackgroundColor
}
|
ImageSmall |
Component {
Width
Height
Crop
DoNotUpscale
FillCanvas
Compression
Quality
Format
BackgroundColor
}
|
ImageMedium |
Component {
Width
Height
Crop
DoNotUpscale
FillCanvas
Compression
Quality
Format
BackgroundColor
}
|
DisableImageEngine | System.Boolean |
DisableLazyLoad | System.Boolean |
FilterPrimary |
|
FilterSecondary |
|
FilterColor | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Image { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Image { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderLink
Settings
Target |
|
Rel |
|
Download | System.Boolean |
Id | System.String |
Title | System.String |
AltText | System.String |
OnClick | System.String |
CssClass | System.String |
Disabled | System.Boolean |
Icon |
Component {
Prefix
Name
Color
CssClass
LabelPosition
Label
HelperName
}
|
Name | System.String |
Href | System.String |
ButtonLayout |
|
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Link { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Link { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderModal
My text
To trigger a modal, simply force the associated checkbox to true with javascript. E.g. document.getElementById('ModalTestModalTrigger').value = true;
Settings
Id | System.String |
Heading |
Component {
Title
Level
Color
Link
CssClass
Icon
IconPosition
HelperName
}
|
Width |
|
Height |
|
BodyText | System.String |
OnClose | System.String |
BodyTemplate | |
DisableDarkOverlay | System.Boolean |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Modal { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Modal { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderNotificationMessage
Settings
Id | System.String |
Message | System.String |
MessageType |
|
MessageLayout |
|
CssClass | System.String |
Icon |
Component {
Prefix
Name
Color
CssClass
LabelPosition
Label
HelperName
}
|
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new NotificationMessage { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new NotificationMessage { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderNumberField
Settings
Min | System.Nullable`1[System.Int32] |
Max | System.Nullable`1[System.Int32] |
Step | System.Int32 |
ReadOnly | System.Boolean |
OnKeyUp | System.String |
OnInput | System.String |
OnFocus | System.String |
ActionButton |
Component {
ConfirmText
ConfirmTitle
ButtonType
Link
Target
IconPosition
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Value | |
Name | System.String |
Id | System.String |
Label | System.String |
HelpText | System.String |
Link |
Component {
Target
Rel
Download
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Value | System.String |
Disabled | System.Boolean |
Required | System.Boolean |
ErrorMessage | System.String |
CssClass | System.String |
OnChange | System.String |
OnClick | System.String |
WrapperCssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new NumberField { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new NumberField { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderRating
Settings
Score | System.Int32 |
OutOf | System.Int32 |
Type |
|
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Rating { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Rating { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderSelectField
Settings
Default |
Component {
Id
Checked
Disabled
Value
Label
ExtraAttributes
Type
Name
OnChange
OnClick
ErrorMessage
HelperName
}
|
Options |
Component {
Capacity
Count
Item
}
|
ActionButton |
Component {
ConfirmText
ConfirmTitle
ButtonType
Link
Target
IconPosition
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Name | System.String |
Id | System.String |
Label | System.String |
HelpText | System.String |
Link |
Component {
Target
Rel
Download
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Value | System.String |
Disabled | System.Boolean |
Required | System.Boolean |
ErrorMessage | System.String |
CssClass | System.String |
OnChange | System.String |
OnClick | System.String |
WrapperCssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new SelectField { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new SelectField { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderSticker
On sale
Settings
Title | System.String |
Color | System.String |
BackgroundColor | System.String |
Style |
|
Size |
|
CssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Sticker { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Sticker { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderStickersCollection
On sale
New
Black friday
Settings
Stickers |
Component {
Capacity
Count
Item
}
|
CssClass | System.String |
Position |
|
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new StickersCollection { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new StickersCollection { OPTIONAL_settings })
Markup result
Helper method: RenderTable
Color | Blue |
Size | M |
Settings
Id | System.String |
CssClass | System.String |
Design |
|
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Table { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Table { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderTable
Meat | Proteins (g) | Fats (g) | Carbs (g) |
Chicken | 20,8 | 8,8 | 0,6 |
Pork | 19,4 | 7,1 | 0 |
Beef | 18,9 | 12,4 | 0 |
CustomCell | CustomCell2 | ||
---|---|---|---|
string1 | string2 | ||
1 | 2 | 3 | 4 |
Settings
Id | System.String |
CssClass | System.String |
Design |
|
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Table { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Table { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderText
My text
Settings
Content | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new Text { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new Text { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderTextareaField
Settings
Placeholder | System.String |
MaxLength | System.Int32 |
Rows | System.Int32 |
ReadOnly | System.Boolean |
OnKeyUp | System.String |
OnInput | System.String |
OnFocus | System.String |
Name | System.String |
Id | System.String |
Label | System.String |
HelpText | System.String |
Link |
Component {
Target
Rel
Download
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Value | System.String |
Disabled | System.Boolean |
Required | System.Boolean |
ErrorMessage | System.String |
CssClass | System.String |
OnChange | System.String |
OnClick | System.String |
WrapperCssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Helper method: RenderTextField
Settings
Type |
|
Placeholder | System.String |
MaxLength | System.Int32 |
ReadOnly | System.Boolean |
OnKeyUp | System.String |
OnInput | System.String |
OnFocus | System.String |
ActionButton |
Component {
ConfirmText
ConfirmTitle
ButtonType
Link
Target
IconPosition
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Name | System.String |
Id | System.String |
Label | System.String |
HelpText | System.String |
Link |
Component {
Target
Rel
Download
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Value | System.String |
Disabled | System.Boolean |
Required | System.Boolean |
ErrorMessage | System.String |
CssClass | System.String |
OnChange | System.String |
OnClick | System.String |
WrapperCssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new TextField { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new TextField { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderAddToCart
Settings
WrapperCssClass | System.String |
Disabled | System.Boolean |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new AddToCart { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new AddToCart { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderAddToCartButton
Settings
HideTitle | System.Boolean |
ProductId | System.String |
VariantId | System.String |
UnitId | System.String |
BuyForPoints | System.Boolean |
ProductInfo | System.String |
QuantitySelectorId | System.String |
ConfirmText | System.String |
ConfirmTitle | System.String |
ButtonType |
|
Link | System.String |
Target |
|
IconPosition |
|
Id | System.String |
Title | System.String |
AltText | System.String |
OnClick | System.String |
CssClass | System.String |
Disabled | System.Boolean |
Icon |
Component {
Prefix
Name
Color
CssClass
LabelPosition
Label
HelperName
}
|
Name | System.String |
Href | System.String |
ButtonLayout |
|
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new AddToCartButton { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new AddToCartButton { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderQuantitySelector
Settings
Min | |
Max | |
Step | |
Min | System.Nullable`1[System.Int32] |
Max | System.Nullable`1[System.Int32] |
Step | System.Int32 |
ReadOnly | System.Boolean |
OnKeyUp | System.String |
OnInput | System.String |
OnFocus | System.String |
ActionButton |
Component {
ConfirmText
ConfirmTitle
ButtonType
Link
Target
IconPosition
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Value | |
Name | System.String |
Id | System.String |
Label | System.String |
HelpText | System.String |
Link |
Component {
Target
Rel
Download
Id
Title
AltText
OnClick
CssClass
Disabled
Icon
Name
Href
ButtonLayout
ExtraAttributes
HelperName
}
|
Value | System.String |
Disabled | System.Boolean |
Required | System.Boolean |
ErrorMessage | System.String |
CssClass | System.String |
OnChange | System.String |
OnClick | System.String |
WrapperCssClass | System.String |
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new QuantitySelector { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new QuantitySelector { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderUnitSelector
Select unit
Piece
Test unit
Settings
Id | System.String |
OptionsContent | System.String |
SelectedOption | System.String |
Disabled | System.Boolean |
CssClass | System.String |
Options |
Component {
Capacity
Count
Item
}
|
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new UnitSelector { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new UnitSelector { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderVariantMatrix
Blue | |
Red | |
0
|
Settings
ProductId | System.String |
Description | System.String |
EnableCounters | System.Boolean |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new VariantMatrix { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new VariantMatrix { OPTIONAL_settings })
Markup result
Helper method: RenderArticleAuthorAndDate
Skrevet
av Me
den 27-03-1984
Settings
Author | System.String |
Date | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleAuthorAndDate { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleAuthorAndDate { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleBanner
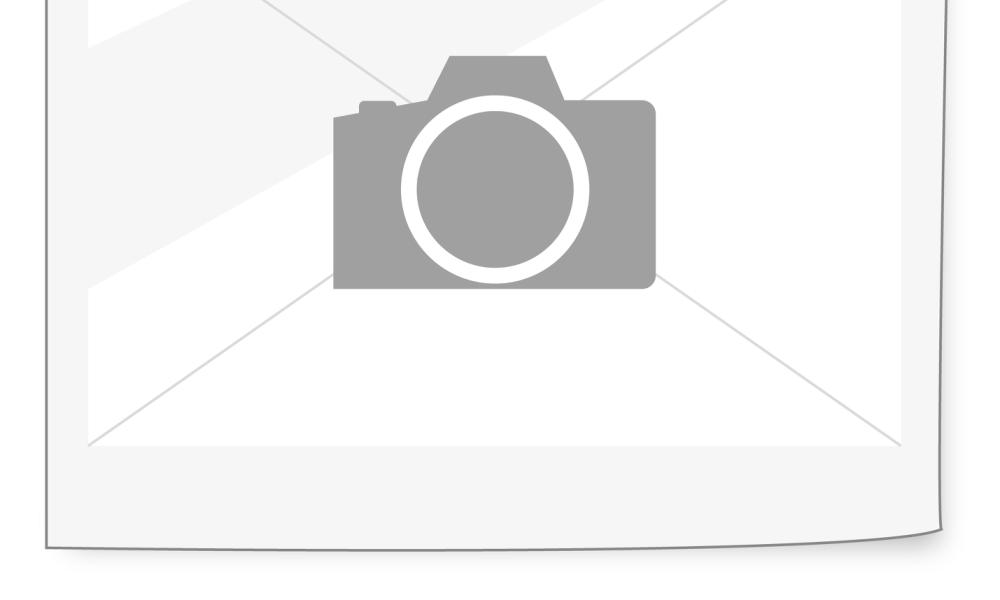
A perfect banner
Settings
UseFilters | System.Boolean |
Layout |
|
Image |
Component {
Path
Title
Id
Link
OnClick
Caption
CssClass
Style
ImageDefault
ImageSmall
ImageMedium
DisableImageEngine
DisableLazyLoad
FilterPrimary
FilterSecondary
FilterColor
ExtraAttributes
HelperName
}
|
Heading | System.String |
Subheading | System.String |
Author | System.String |
Date | System.String |
Category | System.String |
CategoryColor | System.String |
Link | System.String |
LinkText | System.String |
TextLayout |
|
TextColor | System.String |
ButtonLayout |
|
RatingScore | System.Int32 |
RatingOutOf | System.Int32 |
ExternalParagraphId | System.Int32 |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleBanner { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleBanner { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleBodyRow
Settings
TopLayout | System.String |
TextLayout | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleBodyRow { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleBodyRow { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleHeader
Guides
Me 01-01-2020
Settings
Layout |
|
Image |
Component {
Path
Title
Id
Link
OnClick
Caption
CssClass
Style
ImageDefault
ImageSmall
ImageMedium
DisableImageEngine
DisableLazyLoad
FilterPrimary
FilterSecondary
FilterColor
ExtraAttributes
HelperName
}
|
Heading | System.String |
Subheading | System.String |
Author | System.String |
Date | System.String |
Category | System.String |
CategoryColor | System.String |
Link | System.String |
LinkText | System.String |
TextLayout |
|
TextColor | System.String |
ButtonLayout |
|
RatingScore | System.Int32 |
RatingOutOf | System.Int32 |
ExternalParagraphId | System.Int32 |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleHeader { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleHeader { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleImage
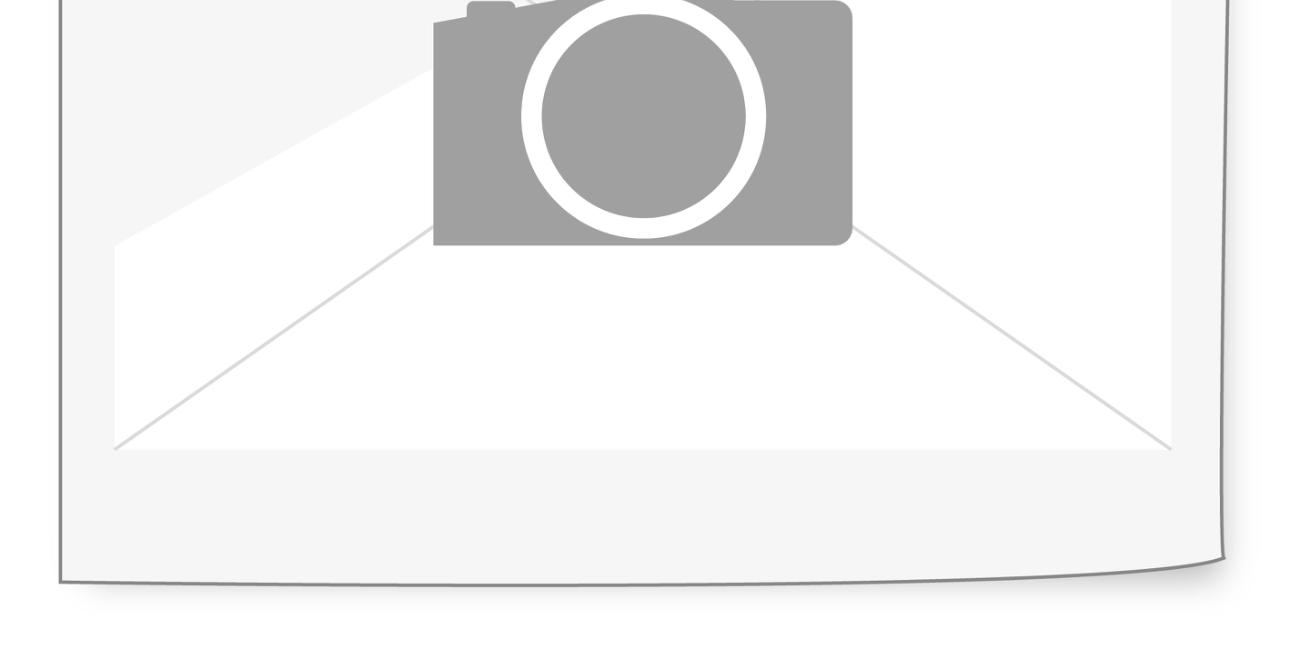
Settings
Image |
Component {
Path
Title
Id
Link
OnClick
Caption
CssClass
Style
ImageDefault
ImageSmall
ImageMedium
DisableImageEngine
DisableLazyLoad
FilterPrimary
FilterSecondary
FilterColor
ExtraAttributes
HelperName
}
|
EnableFullImage | System.Boolean |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleImage { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleImage { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleLink
Settings
ConfirmText | System.String |
ConfirmTitle | System.String |
ButtonType |
|
Link | System.String |
Target |
|
IconPosition |
|
Id | System.String |
Title | System.String |
AltText | System.String |
OnClick | System.String |
CssClass | System.String |
Disabled | System.Boolean |
Icon |
Component {
Prefix
Name
Color
CssClass
LabelPosition
Label
HelperName
}
|
Name | System.String |
Href | System.String |
ButtonLayout |
|
ExtraAttributes | System.Collections.Generic.Dictionary`2[System.String,System.String] |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleLink { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleLink { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleListFilter
Settings
Options | |
Label | System.String |
SystemName | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleListFilter { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleListFilter { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleListSearch
Settings
Title | System.String |
SearchParameter | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleListSearch { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleListSearch { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleMenu
Settings
Title | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleMenu { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleMenu { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleMenuItem
Settings
Title | System.String |
Link | System.String |
Href | System.String |
OnClick | System.String |
Icon | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleMenuItem { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleMenuItem { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleQuote
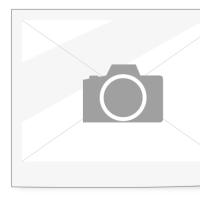
I would like to quote that this is so.
Settings
Image |
Component {
Path
Title
Id
Link
OnClick
Caption
CssClass
Style
ImageDefault
ImageSmall
ImageMedium
DisableImageEngine
DisableLazyLoad
FilterPrimary
FilterSecondary
FilterColor
ExtraAttributes
HelperName
}
|
Text | System.String |
Author | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleQuote { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleQuote { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleSubHeader
My sub header
Settings
Title | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleSubHeader { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleSubHeader { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleSummary
This is a summary
Settings
Text | System.String |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleSummary { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleSummary { OPTIONAL_settings })
Implementation example
Markup result
Helper method: RenderArticleText
Normal body text...
Settings
Text | System.String |
EnableLargeText | System.Boolean |
HelperName | System.String |
Basic usage
Block myBlock = new Block
{
Id = "MyBlock",
SortId = 10,
Component = new ArticleText { OPTIONAL_settings }
};
myPage.Add("MyContainer", myBlock);
Or
@Render(new ArticleText { OPTIONAL_settings })
Implementation example
Markup result